Alright, so today I’m gonna share my experience with “bat spirit” – yeah, it sounds kinda weird, but trust me, it’s a thing (at least in my world).
First off, what even is “bat spirit”? Well, it’s just my silly name for a batch script that automates some boring tasks I gotta do regularly. I’m lazy, okay? If I can make the computer do it, I will.
The Problem: Renaming a bunch of files. I had a folder with like, a million files. Okay, maybe not a million, but a LOT. And they all had these crazy, inconsistent names. Think like “report_*”, “Report_Jan *”, “REPORT_*” – you get the picture. I needed them all to be consistently named, something like “report_*”. Doing it by hand? Forget about it.
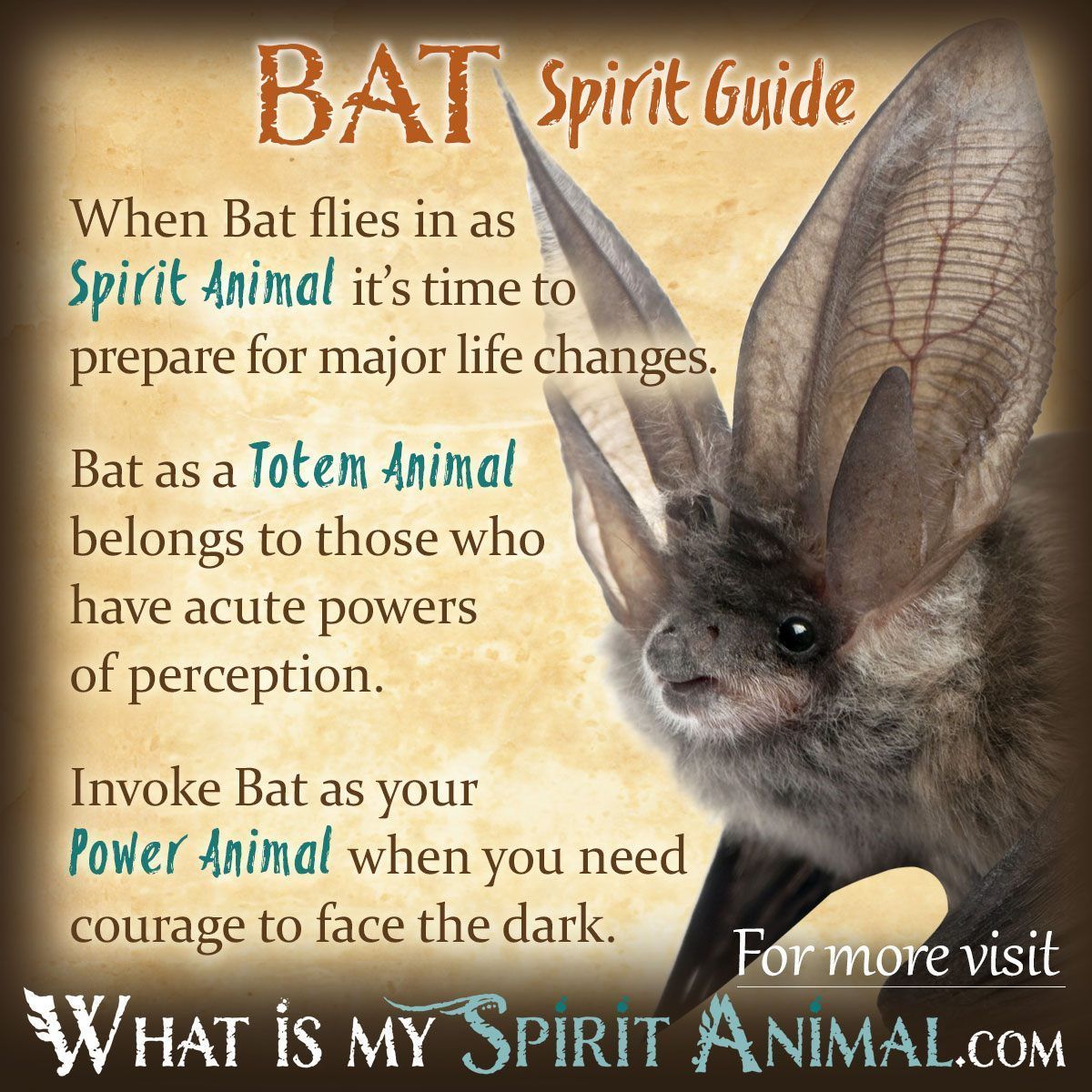
The Plan: Batch script to the rescue! I hadn’t written a batch script in ages, but I figured, how hard could it be? Famous last words, right?
Step 1: Open Notepad (the OG IDE). Yeah, I’m old school. Notepad it is. I fired it up and started thinking about the logic. I knew I needed to loop through the files, grab the date part, and then rename the file.
Step 2: The Looping Magic (FOR loop). First thing was to loop through all the files in the directory.
The code was something like this:
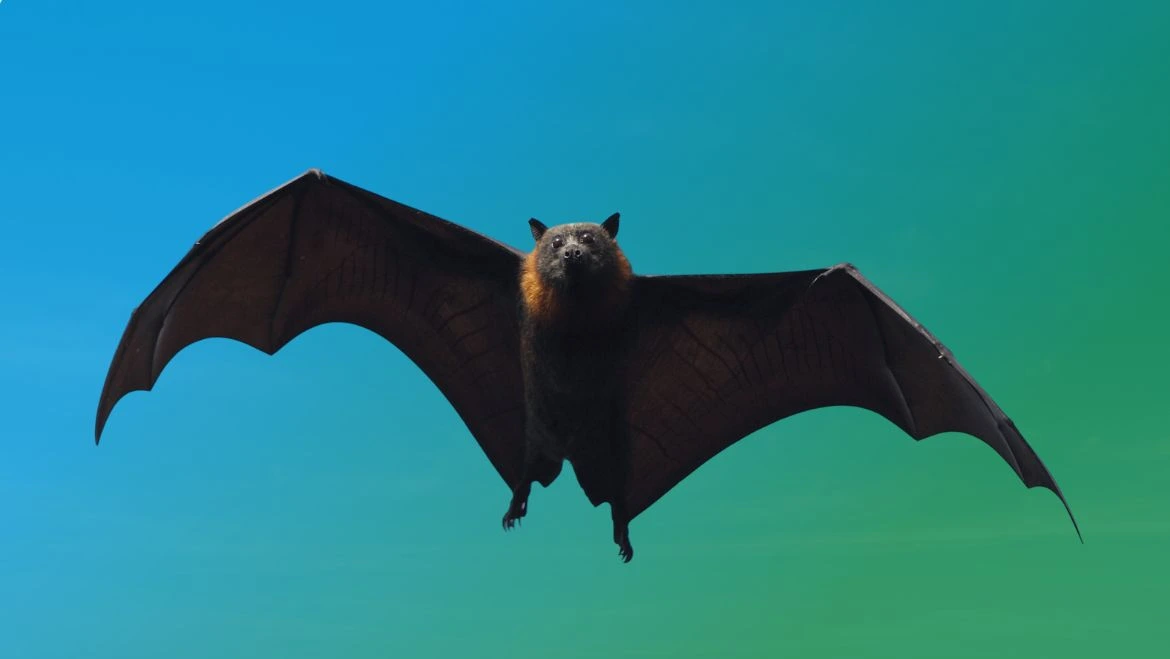
FOR %%A IN (.pdf) DO (
echo "%%A"
Just to make sure it worked, I started with a simple echo
to print the filenames. And boom, it worked! I could see all the .pdf files listed in the command prompt. Small victory, but still a victory.
Step 3: Date Extraction – This was the headache. This is where things got hairy. I had to figure out how to extract the date part from those crazy filenames. After a lot of trial and error (and Googling!), I ended up using some string manipulation tricks with environment variables. Here’s a simplified idea:
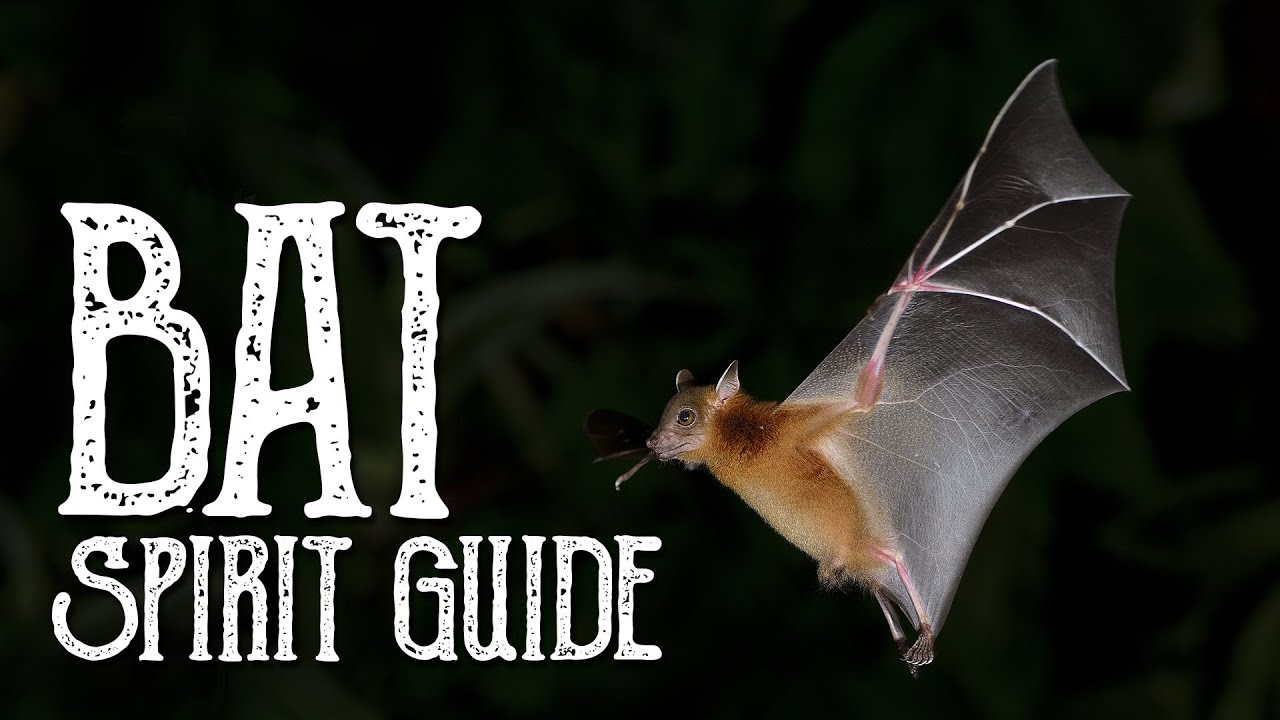
SET filename=%%A
SET datepart=%filename:~7,10%
That %filename:~7,10%
is the key. It’s saying “take the filename, start at the 7th character, and grab the next 10 characters.” It’s not perfect, depends on the file name, some file names are different format. Gotta adjust the numbers.
Step 4: Formatting the Date. Now I had the date, but it was still in the wrong format. More string manipulation! I basically had to break the date into month, day, and year, and then rearrange them. It was ugly, I won’t lie. Lots of substring operations and temporary variables.
Step 5: Renaming the File. Finally! The moment of truth. I used the ren
command to rename the file with the new, consistent name. The whole command looked something like this (after all the date manipulation):
ren "%%A" "report_%year%%month%%day%.pdf"
Step 6: Putting It All Together. I combined all the steps into one big, messy batch script. It was probably the ugliest code I’ve ever written, but hey, it worked! I ran the script, and after a few nervous minutes, all the files were renamed perfectly.
The Result: Victory! (and a slightly cleaner folder). Was it elegant? No. Was it efficient? Probably not. But did it save me hours of manual work? Absolutely. And that’s what matters.
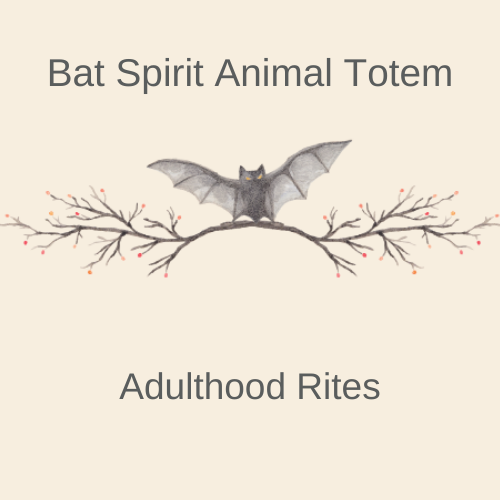
Lessons Learned:
- Batch scripting is still useful for quick and dirty automation.
- String manipulation in batch scripts is a pain, but doable.
- Google is your friend.
- Don’t be afraid to get your hands dirty and write some ugly code if it gets the job done.
So, that’s my “bat spirit” story. Hope you enjoyed it! Now, if you’ll excuse me, I gotta go find something else to automate…