Today, I was messing around with LeetCode, trying to keep my coding skills sharp, you know? I decided to tackle problem 59, “Spiral Matrix II”. It’s all about generating an n x n matrix filled with numbers from 1 to n2 in a spiral order. Sounds kinda cool, right?
So, I started by thinking, “Okay, how would I do this if I were, like, physically filling this matrix?” I’d go right, then down, then left, then up, and repeat, getting closer and closer to the center. That’s the basic idea I wanted to translate into code.
First, I created an empty n x n matrix filled with zeros. Just a placeholder for now.
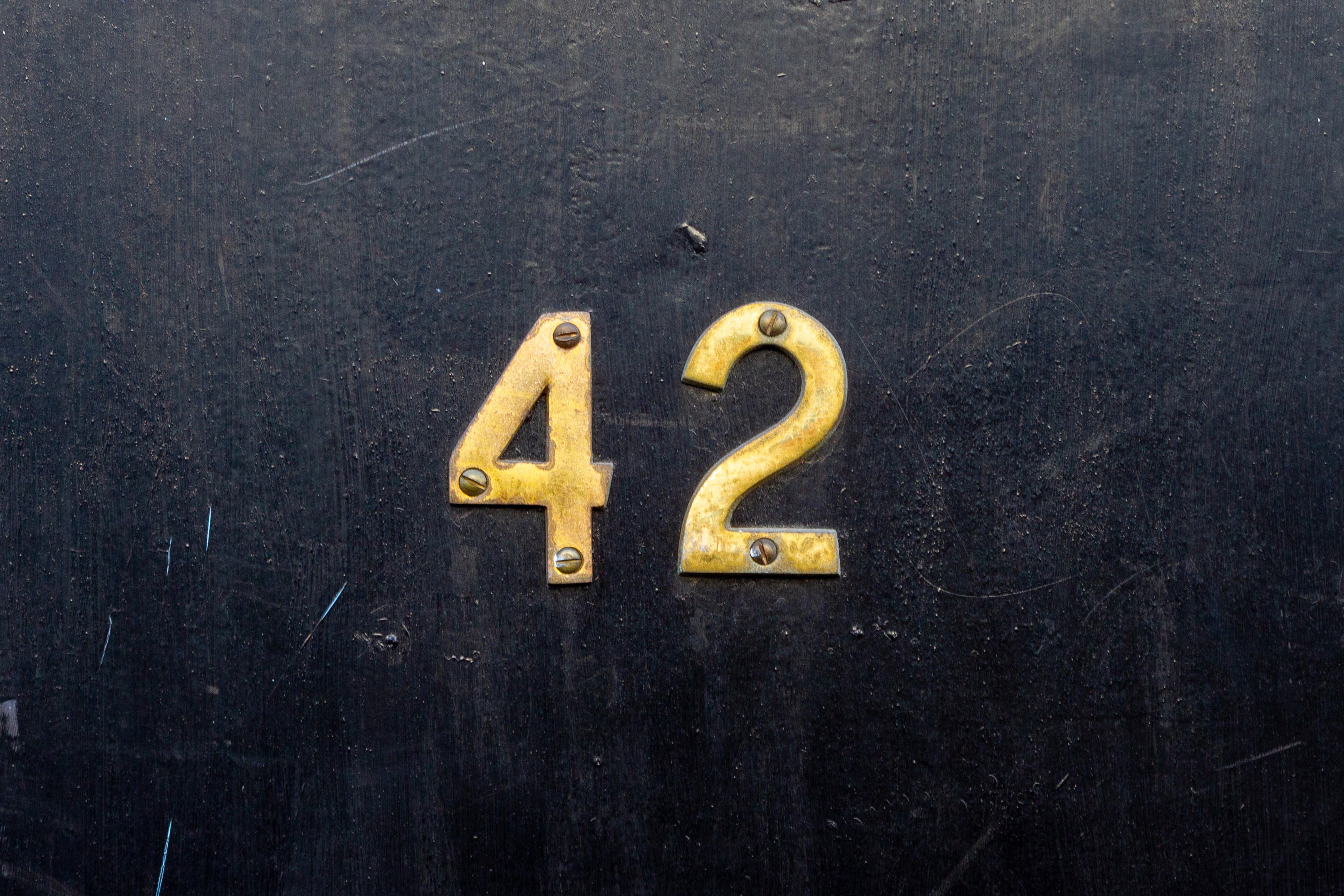
matrix = [[0] n for _ in range(n)]
Then, I set up some variables to keep track of where I am in the matrix: `row_start`, `row_end`, `col_start`, `col_end`. And, of course, a `num` variable to hold the current number I’m placing, starting with 1.
The main part is a `while` loop. It keeps going as long as I haven’t filled the whole matrix. Inside, I have four `for` loops, one for each direction (right, down, left, up).
- Right: I loop from `col_start` to `col_end`, filling the current row with increasing numbers. Then, I increment `row_start` because I’m done with that row.
- Down: I loop from `row_start` to `row_end`, filling the current column. Then, I decrement `col_end`.
- Left: I loop backwards from `col_end` to `col_start`, filling the current row. Then, I decrement `row_end`.
- Up: I loop backwards from `row_end` to `row_start`, filling the current column. Then, I increment `col_start`.
It’s like drawing a spiral with code, one layer at a time. After each of these `for` loops, I update the boundaries, because that part of the matrix is now filled. I also increase number each insert number.
I ran into a couple of tricky things. The first one was getting the loop conditions right. I had to be careful not to go out of bounds or overwrite numbers I’d already placed. Second one is how to design a while loop condition.
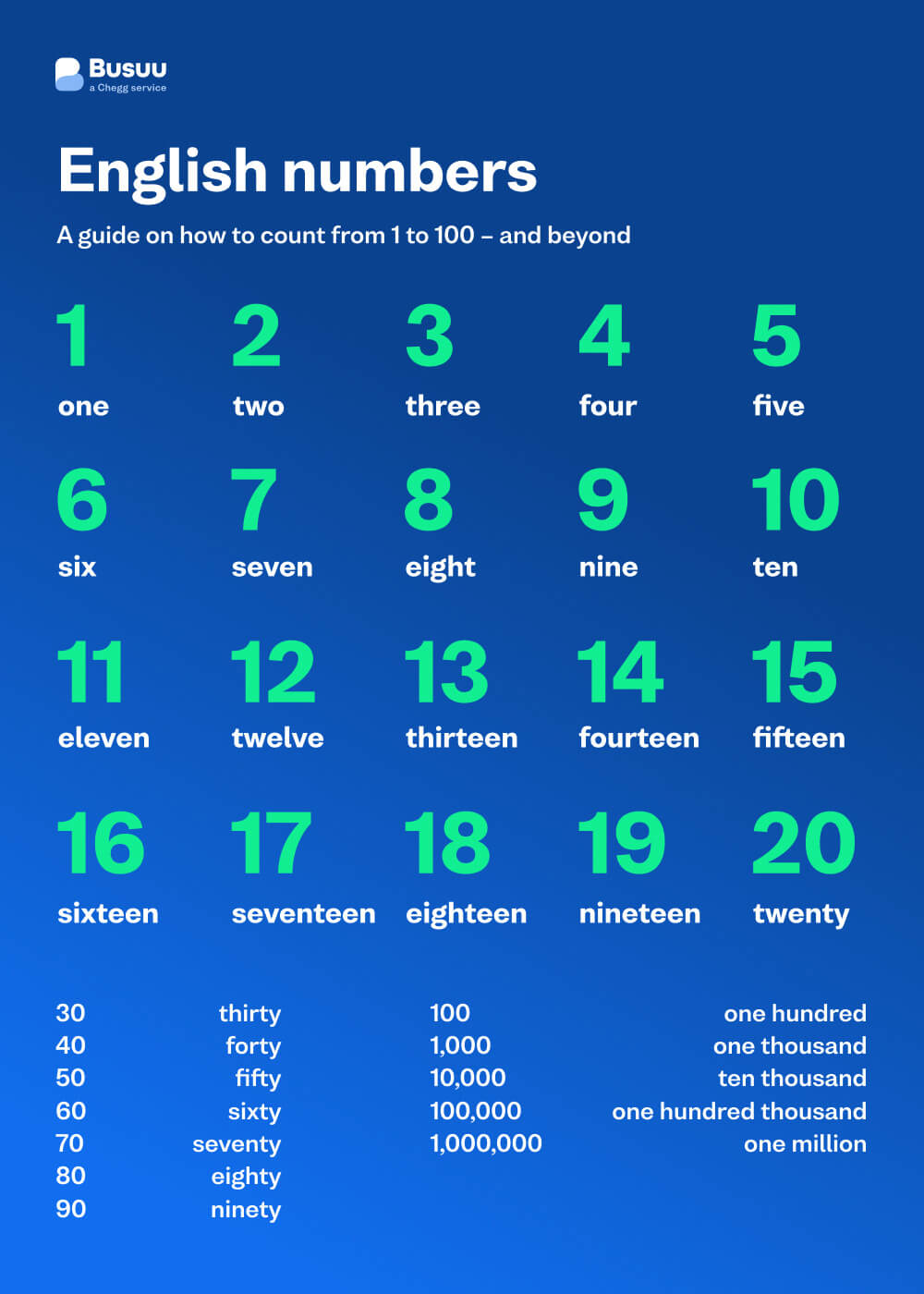
After some trial and error, and probably a few off-by-one errors, I finally got it working! The matrix was spiraling perfectly.
Then I moved to the next test and then input 42, and the generated matrix is still what I am expected. Problem solved.